Send your notifications in minutes
Whether for transactional, engagement or CRM messages, Sweego provides a robust platform to reach your users and customers easily.
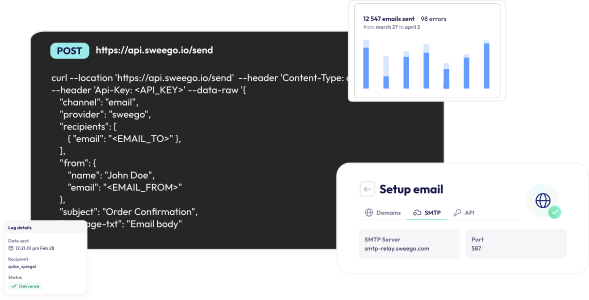
API by developers, for developers
Integrating our API into your product is easy and quick. With our robust RESTful APIs, the integration of notifications is straightforward, whether you’re sending transactional emails, sms or bulk messages.
Curl
Python
Go
PHP
NodeJS
Ruby
C#
Java
PowerShell
curl -L -X POST 'https://api.sweego.io/send' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Api-Key: <API_KEY_VALUE>' \
--data-raw '{
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": false,
"headers": {}
}'
import requests
import json
url = "https://api.sweego.io/send"
payload = json.dumps({
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": False,
"headers": {}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Api-Key': '<API_KEY_VALUE>'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.sweego.io/send"
method := "POST"
payload := strings.NewReader(`{
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": false,
"headers": {}
}`)
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/json")
req.Header.Add("Accept", "application/json")
req.Header.Add("Api-Key", "<API_KEY_VALUE>")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.sweego.io/send',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": false,
"headers": {}
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'Accept: application/json',
'Api-Key: <API_KEY_VALUE>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
const https = require('follow-redirects').https;
const fs = require('fs');
let options = {
'method': 'POST',
'hostname': 'api.sweego.io',
'path': '/send',
'headers': {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Api-Key': '<API_KEY_VALUE>'
},
'maxRedirects': 20
};
const req = https.request(options, (res) => {
let chunks = [];
res.on("data", (chunk) => {
chunks.push(chunk);
});
res.on("end", (chunk) => {
let body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", (error) => {
console.error(error);
});
});
let postData = JSON.stringify({
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": false,
"headers": {}
});
req.write(postData);
req.end();
require "uri"
require "json"
require "net/http"
url = URI("https://api.sweego.io/send")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/json"
request["Accept"] = "application/json"
request["Api-Key"] = "<API_KEY_VALUE>"
request.body = JSON.dump({
"campaign-id": "string",
"channel": "email",
"provider": "string",
"recipients": [
{
"email": "user@example.com",
"name": "string"
}
],
"from": {
"email": "user@example.com",
"name": "string"
},
"subject": "string",
"message-txt": "string",
"message-html": "string",
"template-id": "string",
"variables": {},
"campaign-tag": "string",
"campaign-type": "market",
"dry-run": false,
"headers": {}
})
response = https.request(request)
puts response.read_body
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.sweego.io/send", Method.Post);
request.AddHeader("Content-Type", "application/json");
request.AddHeader("Accept", "application/json");
request.AddHeader("Api-Key", "<API_KEY_VALUE>");
var body = @"{" + "\n" +
@" ""campaign-id"": ""string""," + "\n" +
@" ""channel"": ""email""," + "\n" +
@" ""provider"": ""string""," + "\n" +
@" ""recipients"": [" + "\n" +
@" {" + "\n" +
@" ""email"": ""user@example.com""," + "\n" +
@" ""name"": ""string""" + "\n" +
@" }" + "\n" +
@" ]," + "\n" +
@" ""from"": {" + "\n" +
@" ""email"": ""user@example.com""," + "\n" +
@" ""name"": ""string""" + "\n" +
@" }," + "\n" +
@" ""subject"": ""string""," + "\n" +
@" ""message-txt"": ""string""," + "\n" +
@" ""message-html"": ""string""," + "\n" +
@" ""template-id"": ""string""," + "\n" +
@" ""variables"": {}," + "\n" +
@" ""campaign-tag"": ""string""," + "\n" +
@" ""campaign-type"": ""market""," + "\n" +
@" ""dry-run"": false," + "\n" +
@" ""headers"": {}" + "\n" +
@"}";
request.AddStringBody(body, DataFormat.Json);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"campaign-id\": \"string\",\n \"channel\": \"email\",\n \"provider\": \"string\",\n \"recipients\": [\n {\n \"email\": \"user@example.com\",\n \"name\": \"string\"\n }\n ],\n \"from\": {\n \"email\": \"user@example.com\",\n \"name\": \"string\"\n },\n \"subject\": \"string\",\n \"message-txt\": \"string\",\n \"message-html\": \"string\",\n \"template-id\": \"string\",\n \"variables\": {},\n \"campaign-tag\": \"string\",\n \"campaign-type\": \"market\",\n \"dry-run\": false,\n \"headers\": {}\n}");
Request request = new Request.Builder()
.url("https://api.sweego.io/send")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Api-Key", "<API_KEY_VALUE>")
.build();
Response response = client.newCall(request).execute();
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/json")
$headers.Add("Accept", "application/json")
$headers.Add("Api-Key", "<API_KEY_VALUE>")
$body = @"
{
`"campaign-id`": `"string`",
`"channel`": `"email`",
`"provider`": `"string`",
`"recipients`": [
{
`"email`": `"user@example.com`",
`"name`": `"string`"
}
],
`"from`": {
`"email`": `"user@example.com`",
`"name`": `"string`"
},
`"subject`": `"string`",
`"message-txt`": `"string`",
`"message-html`": `"string`",
`"template-id`": `"string`",
`"variables`": {},
`"campaign-tag`": `"string`",
`"campaign-type`": `"market`",
`"dry-run`": false,
`"headers`": {}
}
"@
$response = Invoke-RestMethod 'https://api.sweego.io/send' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
Not enough time to implement API? Use our SMTP
Host, port, login and password is the only information you need to send email by SMTP
Our features for your notifications
Scalability
Integrate email, slack, sms & other channels into your application easily
Authentication
Protect your sender reputation with SPF & DKIM automatically
Email builder
Use our integrated email builder to create emails templates and send beautiful and compatible emails
Custom Header
Add customized headers to supercharge your statistics & logs
Webhook
Configure event webhooks to automate your workflow with Sweego data
Test Mode
Test your email flow without sending your email to a real address